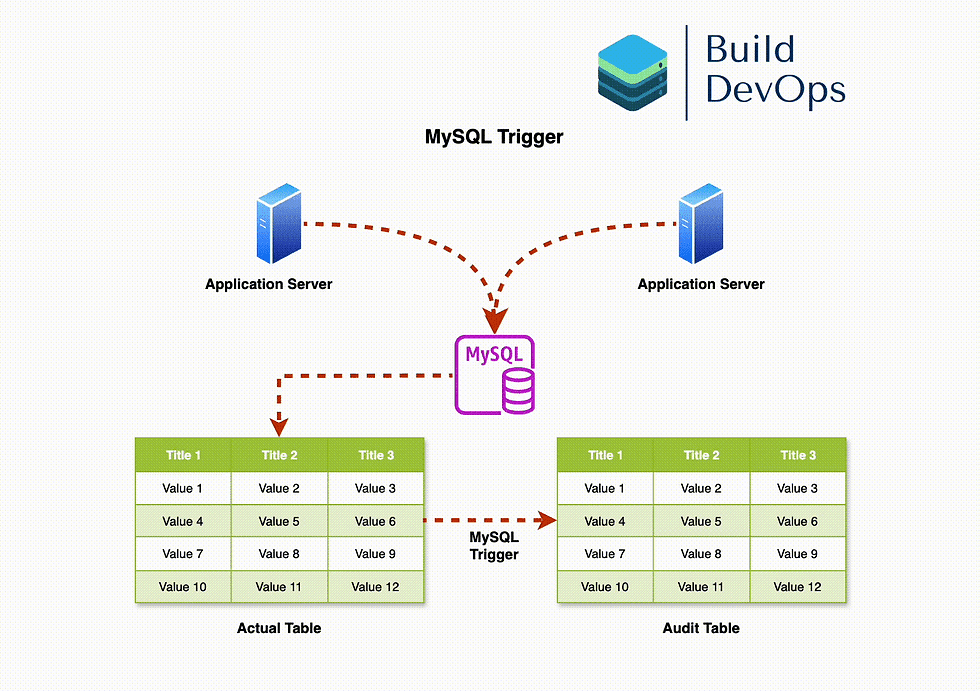
Introduction to MySQL Triggers
In the realm of database management, automation is key to maintaining data integrity and enforcing business rules without manual intervention. One powerful feature that facilitates this automation in MySQL is triggers. Triggers are a type of stored procedure that automatically execute in response to certain events on a particular table. These events can be INSERT, UPDATE, or DELETE operations.
In this blog, we'll explore MySQL triggers in detail, understand their syntax, and see practical examples of how they can be used to streamline database operations.
What is a MySQL Trigger?
A MySQL trigger is a set of SQL statements that automatically executes or fires when a specified event occurs on a table. Triggers can be used to:
Automatically validate or modify data before it is inserted or updated.
Log changes to data for auditing purposes.
Enforce referential integrity across tables.
Synchronize tables.
Syntax of MySQL Triggers
To create a trigger, you need to define the following elements:
Trigger name: A unique identifier for the trigger.
Trigger event: The event that activates the trigger (INSERT, UPDATE, or DELETE).
Trigger timing: Specifies when the trigger activates in relation to the triggering event (BEFORE or AFTER).
Trigger body: The SQL statements to execute when the trigger fires.
Here’s the general syntax for creating a trigger:
CREATE TRIGGER trigger_name
{BEFORE | AFTER} {INSERT | UPDATE | DELETE}
ON table_name
FOR EACH ROW
BEGIN
-- SQL statements
END;
Example 1: Auditing Table Changes
Let’s consider a scenario where we want to track changes to an employees table. We can create an audit_employees table to log these changes and then set up a trigger to insert a record into the audit table whenever an UPDATE occurs on the employees table.
Step 1: Create the Audit Table
First, we create the audit_employees table to store the audit records.
CREATE TABLE audit_employees (
audit_id INT AUTO_INCREMENT PRIMARY KEY,
emp_id INT,
old_salary DECIMAL(10, 2),
new_salary DECIMAL(10, 2),
change_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Step 2: Create the Trigger
Next, we create a trigger that fires AFTER UPDATE on the employees table
CREATE TRIGGER after_employee_update
AFTER UPDATE ON employees
FOR EACH ROW
BEGIN
INSERT INTO audit_employees (emp_id, old_salary, new_salary)
VALUES (OLD.emp_id, OLD.salary, NEW.salary);
END;
Explanation
AFTER UPDATE specifies that the trigger should fire after an UPDATE operation on the employees table.
FOR EACH ROW means the trigger will execute once for each row affected by the UPDATE operation.
Inside the BEGIN...END block, we insert a new record into the audit_employees table, capturing the old and new salary values.
Example 2: Condition Based Rules
Consider a scenario where we want to ensure that no employee's salary is reduced. We can create a BEFORE UPDATE trigger on the employees table to enforce this rule.
Step 1: Create the Trigger
CREATE TRIGGER before_employee_update
BEFORE UPDATE ON employees
FOR EACH ROW
BEGIN
IF NEW.salary < OLD.salary THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Salary reduction is not allowed';
END IF;
END;
Explanation
BEFORE UPDATE specifies that the trigger should fire before an UPDATE operation on the employees table.
Inside the BEGIN...END block, we use an IF statement to check if the new salary is less than the old salary.
If the condition is true, the SIGNAL statement raises an error, preventing the UPDATE operation from proceeding.
Example 3: Synchronizing Tables
Suppose we have two tables, orders and order_summary, and we want to keep the order_summary table updated whenever a new order is inserted into the orders table.
Step 1: Create the Order Summary Table
CREATE TABLE order_summary (
order_id INT PRIMARY KEY,
total_amount DECIMAL(10, 2)
);
Step 2: Create the Trigger
CREATE TRIGGER after_order_insert
AFTER INSERT ON orders
FOR EACH ROW
BEGIN
INSERT INTO order_summary (order_id, total_amount)
VALUES (NEW.order_id, NEW.total_amount);
END;
Explanation
AFTER INSERT specifies that the trigger should fire after an INSERT operation on the orders table.
Inside the BEGIN...END block, we insert a new record into the order_summary table, capturing the order ID and total amount from the orders table.
Managing Triggers
Listing Triggers
You can list all triggers in a database using the SHOW TRIGGERS statement:
SHOW TRIGGERS;
Dropping Triggers
To drop a trigger, use the DROP TRIGGER statement:
DROP TRIGGER trigger_name;
Conclusion
MySQL triggers are a powerful tool for automating database tasks, enforcing business rules, and maintaining data integrity. By understanding how to create and manage triggers, you can enhance the functionality and reliability of your database applications. Whether you're auditing changes, enforcing rules, or synchronizing tables, triggers provide a flexible and efficient way to handle these tasks.
Remember to use triggers judiciously, as they can introduce complexity and potential performance overhead. Always test triggers thoroughly to ensure they perform as expected and do not introduce unintended side effects.
2 則留言